- Published on
Data Structures in Javascript.
- Authors
- Name
- Taiwo Ifedayo
- @_taiwoifedayo
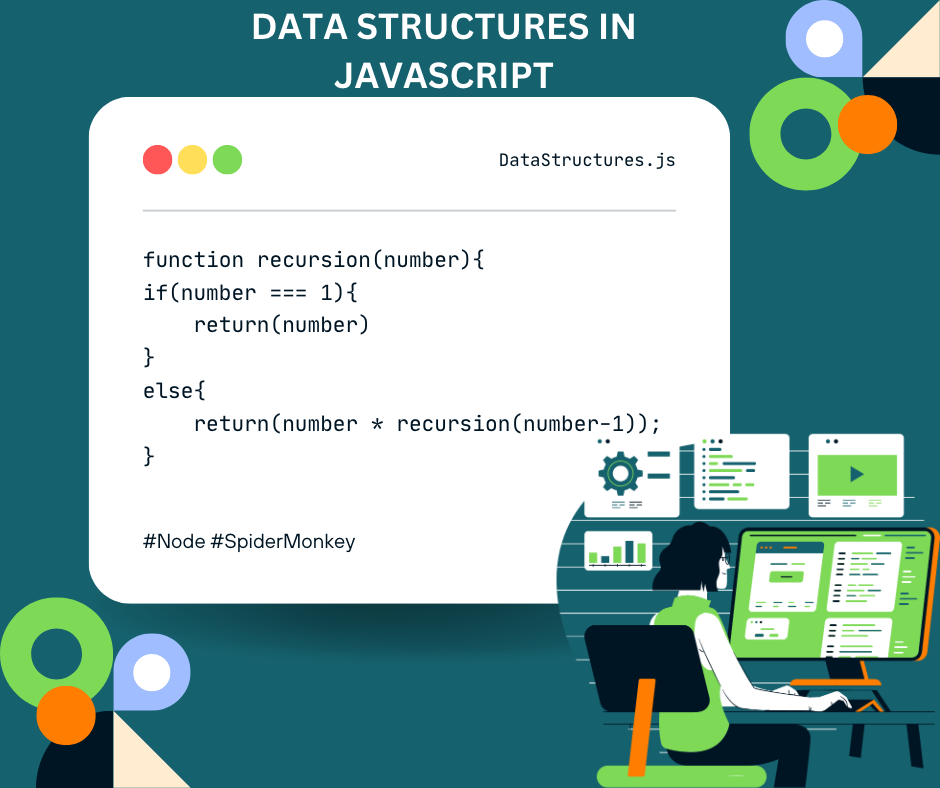
For many years, JavaScript was primarily utilized within web browsers. However, the landscape changed with the advent of platforms like Node and SpiderMonkey.
As JavaScript has transitioned from being solely used for client-side programming, programmers have found a greater need for tools provided by conventional languages such as C++ and Java.
These tools include classic data structures such as linked lists, stacks, queues, and graphs, as well as traditional algorithms for searching and sorting data.
Why Are Data Structures and Algorithms Important?
The title of Niklaus Wirth's programming textbook, "Algorithms + Data Structures = Programs (Prentice-Hall)," encapsulates the essence of computer programming.
Any computer program that goes beyond the trivial "Hello world!" will require some form of data structure to manage the data it manipulates, along with algorithms for transforming the data from input to output.
While many programmers are familiar with the Array data structure, arrays alone may not be sophisticated enough for solving complex problems.
Experienced programmers often acknowledge that once they identify the appropriate data structure, designing and implementing the required algorithms becomes easier.
An example of a data structure that leads to efficient algorithms is the binary search tree (BST). A BST is designed to facilitate finding the minimum and maximum values of a data set, resulting in an algorithm that is more efficient than the best search algorithms available. Programmers unfamiliar with BSTs may opt for simpler data structures that end up being less efficient.
Studying algorithms is crucial because there is usually more than one algorithm that can solve a problem, and knowing the efficient ones is essential for a productive programmer.
Example
- While there are numerous ways to sort a list of data, understanding that the Quicksort algorithm is more efficient than the selection sort algorithm can significantly improve the sorting process.
- Implementing a sequential or linear search algorithm for a list of data is relatively straightforward, but being aware that the binary search algorithm can be twice as efficient as a sequential search will result in a better program.
The study of data structures and algorithms not only teaches which ones are most efficient, but also helps in deciding which data structures and algorithms are most appropriate for a given problem.
When writing a program, trade-offs are inevitable, especially in JavaScript. Familiarity with the various data structures and algorithms covered in this book will enable you to make informed decisions for any programming problem you're trying to solve.
Credits:
Image created with Canva
References from the book Data Structures and Algorithms with JavaScript by Michael McMillan